API Endpoints: Structure, and Key Concepts
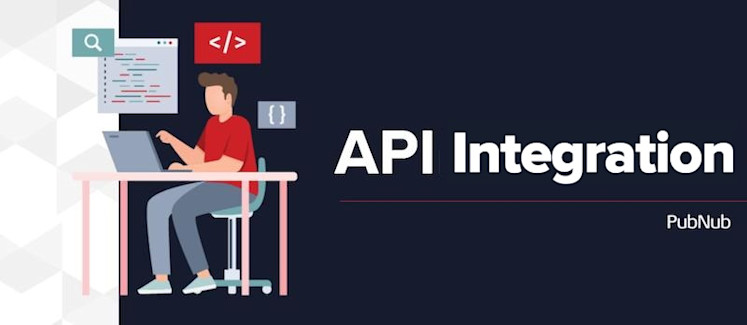
What is an API Endpoint?
An API endpoint is a specific URL or IP address that allows developers to access a particular function or dataset from an application via an Application Programming Interface. Endpoints serve as communication points, exposing functionalities or data from a service to other applications, enabling integration and data exchange across systems. This post will cover the essential components, technical specifics, and key performance benchmarks relevant to API endpoints.
Key Components of an API Endpoint
Address Structure: The base URL of an API endpoint typically begins with the domain or IP address of the server hosting the API, followed by a path that specifies the resource or action.
Example:
https://api.example.com/v1/users
Here, https://api.example.com
is the base URL, /v1
denotes the API version, and /users
specify the endpoint for user-related actions.
HTTP Methods each endpoint uses specific HTTP methods to define the action (GET, POST, DELETE)
Request Parameters different parameter types allow more control over requests:
Path Parameters: Embedded in the endpoint path (e.g., /users/{user_id}).
Query Parameters: Key-value pairs appended to the URL (e.g., /users?age=25).
Body Parameters: Used with POST and PUT requests to send structured data (e.g., JSON payloads).
Response Format is commonly JSON, though XML or other formats may be used. JSON provides a lightweight, readable structure with key-value pairs representing data.
Technical Specifics for Optimized API Endpoints
API Endpoint Authentication and Security Authentication can be implemented using keys, OAuth tokens, or JWTs (JSON Web Tokens). SSL/TLS encryption is essential to secure sensitive data, especially personal or financial information.
Versioning Best practice is to version API endpoints (e.g., /v1/) so changes in structure or functionality don’t disrupt existing integrations.
Pagination and Rate Limiting for large datasets, use pagination (e.g., limit and offset parameters) to control data volume. Rate limiting (e.g., 100 requests per minute) prevents server overload and ensures fair access.
Error Handling A well-designed app interface should provide clear error messages and HTTP status codes (e.g., 404 Not Found, 500 Internal Server Error) to help users troubleshoot.
Endpoint Performance Benchmarks
To ensure optimal API endpoint performance, specific benchmarks and metrics are monitored:
Response Time: Latency, response times should be below 200ms for general requests and under 100ms for latency-sensitive applications (e.g., financial or real-time apps).
Throughput (Requests per Second): Determines the API’s capacity for handling concurrent requests. High-throughput APIs can manage thousands of requests per second, critical for applications with large user bases.
Error Rate Monitors the percentage of failed requests. Maintaining an error rate below 1% is a common benchmark.
Latency Measures the delay in data transmission, affected by factors like geographic distance and network conditions. Keeping good latency (under 120ms) improves user experience.
Data Transfer Size: To optimize payload size, use efficient data formats (e.g., JSON) and avoid unnecessary fields. This is particularly important for mobile applications with limited bandwidth.
API Versioning
APIs evolve as applications grow, often requiring new fields, endpoint deprecation, or structural changes. Versioning provides a way to manage these changes gracefully, ensuring:
Backward Compatibility: Older clients continue to function.
Incremental Improvement: New versions can be introduced without rewriting the entire API.
Controlled Deprecation: Deprecated endpoints can be phased out over time.
Key Approaches to API Versioning
URI Path Versioning (URL Versioning):
Example: /v1/users and /v2/users.
Pros: Simple and explicit; easy to document.
Cons: Tightly coupled with URL, requiring new endpoints for transitions.
Query Parameter Versioning:
Example: /users?version=1.
Pros: Maintains a consistent URL structure.
Cons: Less visible and can be less effective for caching by proxies.
Header Versioning:
Example:
GET /users
Headers:
X-API-Version: 1
Pros: Clean URL structure, versioning abstracted to headers.
Cons: More challenging to document and manage.
Accept Header or Content-Type Versioning:
Example:
GET /users
Headers
Accept: application/vnd.example.v1+json
Pros: Maintains a clean URL structure.
Cons: Adds complexity, particularly for managing multiple formats.
Choosing the Right Versioning Strategy
Path Versioning: Ideal for public APIs due to visibility and simplicity.
Header Versioning: Better for internal or complex APIs where clients have header control.
Accept Header: Useful for APIs with diverse response formats, as it allows for fine-grained control over both version and response type.
Best Practices for API Versioning
Gradual Deprecation: Provide clients with a deprecation timeline.
Avoid Breaking Changes: Try not to disrupt existing functionality. For example, make new fields optional.
Clear Documentation: Maintain separate documentation for each version, including changes and deprecated functionality.
Endpoint Identification
Endpoint identification is the process of defining the address structure (URL or IP), HTTP method, and purpose for each API endpoint, which is crucial to effective API design.
Endpoint Identification Checklist
To ensure thorough endpoint identification:
Are endpoints resource-based (using nouns) and logically structured?
Is naming consistent (plural nouns, lowercase)?
Are HTTP methods correctly aligned with REST principles?
Are parameters (path, query) meaningful and descriptive?
Is the endpoint structure simplified and ready for future versions?
Example: Endpoint Identification in a RESTful API
User-Related Endpoints:
Get all users:
GET /v1/users
Get a specific user:
GET /v1/users/{userId}
Create a new user:
POST /v1/users
Update a user:
PUT /v1/users/{userId}
Delete a user:
DELETE /v1/users/{userId}
Nested Endpoints:
Get books by author: GET /v1/authors/{authorId}/books
API endpoint max payload
The maximum payload size that an API endpoint can handle depends on various factors, including server configuration, network considerations, and API-specific limitations.
Common Size Limits
Typical Limits: Most APIs accept payloads up to 1 MB by default. Common thresholds include 2 MB, 5 MB, and 10 MB.
High-Volume APIs: For APIs dealing with media files (images, videos), the limit might be set higher (e.g., 50 MB or more), but these limits are carefully managed due to server load concerns.
Configuration Constraints
Server Configuration: The server’s configuration (like NGINX or Apache) often restricts payload size. For example:
API Gateway: Many managed API gateways (like AWS API Gateway, Azure API Management) impose limits. AWS, for instance, limits payloads to 10 MB for REST APIs.
Web Server Defaults: Some web servers have lower defaults, such as 2 MB or 5 MB.
HTTP Protocol API endpoint limits
HTTP does not strictly limit request size, but client and server configurations often set constraints to avoid excessive resource use.
Security and Performance Considerations
Large Payloads can slow down API response times and increase latency.
API Security: Large payloads may expose servers to DDoS attacks or other resource-intensive exploits. Setting size limits helps mitigate these risks.
Timeouts: A large payload might cause request timeouts, especially over slower networks.
Workarounds for Large Payloads
Chunking and Streaming: Large data can be divided into chunks or streamed to handle sizes beyond the limit.
File Uploads: For APIs handling large files, consider uploading to an external storage service (like AWS S3) and returning only a reference URL in the API.
Checking the Max Payload Limit for Your API
Check the documentation or API gateway settings to confirm the payload limits.
Test the API endpoint by incrementally increasing payload size until a 413 Payload Too Large
error (or equivalent) is encountered. By adhering to payload size guidelines and considering performance, security, and scalability, you can optimize API endpoints to handle data efficiently and reliably.
Effective API endpoint design enhances API usability, performance, and scalability. By following principles of endpoint identification, implementing versioning strategies, and setting technical benchmarks, you can ensure a robust and reliable API.