An Introduction to Building Realtime Apps with Socket.IO
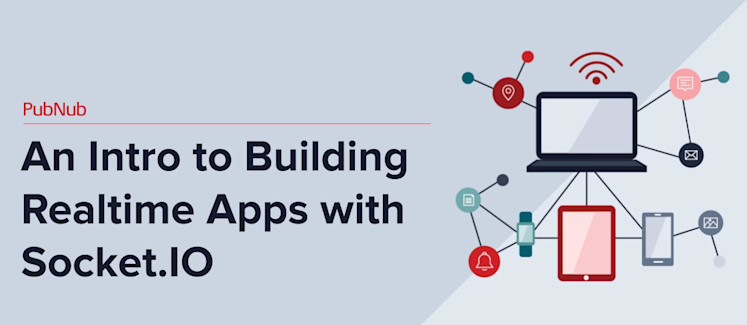
Introduction
More and more businesses are leveraging real-time chat applications and video conferencing to send messages and power customer interactions. As customers embrace these new technologies, app and web development teams must ensure applications can support increasing demand. But that’s easier said than done—numerous performance (like latency) and security issues come with unregulated traffic spikes.
Open-source libraries like Socket.io make it easier for dev teams to build realtime capabilities. However, socket infrastructure isn’t reliable when managing increasing workloads. So, how can dev teams ensure applications can meet growing demands in realtime?
In Part I of this Socket.io blog series, we'll start by understanding what it means to scale your application and briefly introduce you to the world of Socket.io.
What is scalability?
Scalability is a system’s ability to adapt its performance to changes in the number of users, transactions, or data volume. It allows systems to handle two scenarios efficiently:
You can scale up to support new requirements when your workload increases. Your application’s performance remains consistent, and customers can enjoy high-quality user experiences.
When your workload decreases, you can scale down so the application uses only the required resources and runs cost-effectively.
Challenges of scaling real-time applications
Scaling apps like a real-time chat app, chat room, or multiplayer game comes with challenges.
Performance issues
Scaling up allows your system to manage increasing workloads and adds additional server load. Estimating workloads accurately enables dev teams to evaluate if the current server architecture can support the extra workload.
Infrastructure limitations
As the number of users increases, your app may underperform. Available resources may not be able to support the surge in demand. Ensure your infrastructure can handle the expanding workload to offer exceptional user experiences.
Data management challenges
Effective data management fuels real-time capabilities. Design your system to easily scale up to handle increasing data volumes with more users and requests.
Increased costs
Scalability can increase application development costs. Addressing all the above challenges adds to the overall cost, considering investments in new infrastructure, hardware, software, and labor.
Benefits of a scalable real-time system
The benefits scalable real-time systems offer make them worth the investment.
Improved convenience
Developers can save time on additional development by designing the system to scale server capacity to meet workload requirements with just a few clicks.
Increased speed and flexibility
Scalability allows real-time applications to respond faster to spikes in demand. Extra resources are only used when needed.
Reduced cost
Although implementing scalable solutions can be expensive, you can only design your applications to scale when necessary. This way, you only pay for what you use and avoid purchasing extra equipment.
What is Socket.io?
Created in 2010, Socket.io is an open-source library facilitating full-duplex, bi-directional communication between web clients and servers comprising a Node.js server (like Server.js) and a Javascript library for the browser. It’s very similar to WebSockets as it builds on the WebSockets protocol, providing additional capabilities like automatic reconnection and fallback to HTTP long polling. Although most browsers today support WebSockets, Socket.io is still viable. So, even if you decide to use WebSockets for your web application’s backend, you’ll eventually need to implement features like reconnection, acknowledgments, and broadcasting.
Key features of Socket.io
Socket.io offers battle-tested features for creating reliable real-time applications.
HTTP long-polling fallback
HTTP long polling is a variation of standard polling. It duplicates an HTTP server, more efficiently pushing messages to a client (or browser). The Socket.io framework first tries to establish a long polling connection and then tries to upgrade to a WebSocket connection if possible. It uses this approach because establishing a WebSocket connection isn’t guaranteed with firewalls and antivirus software in play.
Automatic reconnection
WebSockets can interrupt the connection under particular conditions without the client and the server knowing. Socket.io works around this by including a heartbeat mechanism that periodically checks the connection status. If the Socket.IO client gets disconnected, it automatically reconnects on the server-side and uses a back-off delay to prevent multiple reconnection attempts.
Packet buffering
If the server disconnects from the server, Socket.io automatically continues buffering events and sends them when the client reconnects. However, this could result in a huge spike in events. Using connected attributes for the client-side Socket instance and volatile events, you can prevent this.
Acknowledgments
Socket.io offers a request-response application programming interface (API) called Acknowledgements. It is a convenient way to send an event and receive a response. You can even design custom responses to events and add request timeouts to make the process more efficient.
Broadcasting
As the name suggests, Broadcasting is the process of sending an event to all connected clients or a subset of clients. This also works when scaling to multiple Socket.io servers.
Multiplexing
Multiplexes (called Namespaces) allow you to split the logic of your application over a single shared connection. It is useful when creating channels only select users can access, like admin or membership channels. Each multiplex will have its event handlers emitters and event listeners), rooms, and middleware.
How does Socket.io work?
Socket.io uses two transport methods to enable socket connections:
HTTP long-polling
WebSockets
Depending on browser and network capabilities, Socket.io automatically selects the best option. But usually, the HTTP long-poll connection is established first, followed by the WebSocket connection.
NOTE: Socket.io goes with HTTP long polling first because, although more popular, WebSockets aren’t supported on all browsers. So, long-polling is done first to guarantee successful connections and reduce load times. When the WebSockets connection is established, HTTP long-polling will act as the fallback so you can confidently continue delivering great real-time experiences.
Socket.io framework
The Socket.io codebase is divided into two distinct layers: Engine.io and the Socket.io protocol. Engine.io is the underlying transport layer used by Socket.IO. It’s a realtime bidirectional communication engine that enables a persistent connection between the server and the client. Engine.io provides the foundation for Socket.IO’s real-time capabilities by handling the low-level communication protocol and managing the connection between the client and the server.
Engine.io uses various transport mechanisms, such as WebSocket, HTTP long-polling, and AJAX, to ensure reliable and efficient client and server communication. It automatically selects the most suitable transport mechanism based on the capabilities of the client and the server.
WebSocket provides a full-duplex communication channel over a single TCP connection. This allows for real-time bidirectional communication with low latency and overhead. In cases where WebSocket is not supported or available, Engine.io falls back to HTTP long-polling, which involves the client continuously sending requests to the server and the server holding the response until new data is available. This allows for real-time communication even in environments where WebSocket is not supported, such as older web browsers or restrictive network configurations.
AJAX is another fallback mechanism used by Engine.io. It involves sending asynchronous HTTP requests from the client to the server regularly to check for new data. While AJAX-based communication is not as efficient as WebSocket or long-polling, it can still provide a basic level of real-time functionality in situations where other mechanisms are unavailable. The Socket.io protocol delivers additional features (automatic reconnection, packet buffering, acknowledgments, etc.) using the communication channel established by Engine.io. However, you need to host and maintain your cluster of servers when building out your Socket.io application.
Common Socket.io commands
The Socket.io library provides a wide range of functionality for real-time communication, including various commands for event handling, room management, and more.
Some common commands used with Socket.io are:
io.on('connection', callback)
: This command is used on the server-side to listen for new client connections. It triggers the callback function when a new client connects to the server.socket.emit('event', data)
: This command is used on both the server-side and client-side to emit an event to the connected clients. It sends the specified data along with the event to the recipients.io.emit('event', data)
: This command is used on the server-side to emit an event to all connected clients. It sends the specified data along with the event to all clients.socket.on('event', callback)
: This command is used on both the server-side and client-side to listen for a specific event. It triggers the callback function when the specified event is received.socket.broadcast.emit('event', data)
: This command is used on the server-side to emit an event to all connected clients except the sender. It sends the specified data along with the event to all clients except the one who triggered the event.socket.join('room')
: This command is used on the server-side to make a client join a specific room. It allows for grouping clients into different rooms for targeted communication.socket.leave('room')
: This command is used on the server-side to make a client leave a specific room that it had previously joined.io.to('room').emit('event', data)
: This command is used on the server-side to emit an event to all clients in a specific room. It sends the specified data along with the event to all clients in the room.
Part II of this series will explore architectural patterns, considerations, and best practices.
We assist developers in building real-time interactivity for use cases like IoT, web apps, and mobile devices. The platform runs on our edge messaging network, providing customers with the industry's largest and most scalable global infrastructure for interactive applications. With over 15 points of presence worldwide supporting 800 million monthly active users, hundreds of millions of chat messages, and 99.999% reliability, you’ll never have to worry about outages, concurrency limits, or any latency issues caused by traffic spikes. By leveraging our infrastructure, APIs, SDKs, extensive library of step-by-step tutorials, and GitHub, developers can focus on creating innovative and engaging user experiences.
Sign up for a free trial and get up to 200 MAUs or 1M total transactions per month included.